Angular Styling Secrets: How to Use :host and :host-context Like a Pro
Styling Angular components can be tricky, especially with encapsulated styles. But :host and :host-context let you target a component’s root element and adapt styles based on its context—without global CSS hacks. In this guide, you'll learn how to apply, modify, and control styles using these selectors, making your components smarter and more flexible. Let’s dive in!
Understanding :host and :host-context
These selectors originate from Web Components, where encapsulated styling is essential.
Angular’s ViewEncapsulation mechanism uses the Shadow DOM, or at least emulates it, to scope component styles.
:host lets us style the component’s root element.
And :host-context lets us apply styles based on the context of something higher up in the DOM tree.
Let’s look at how and why we may want to use them.
Using :host: Styling the Component’s Root Element
Here we have a simple application showing a basic sign-up form:
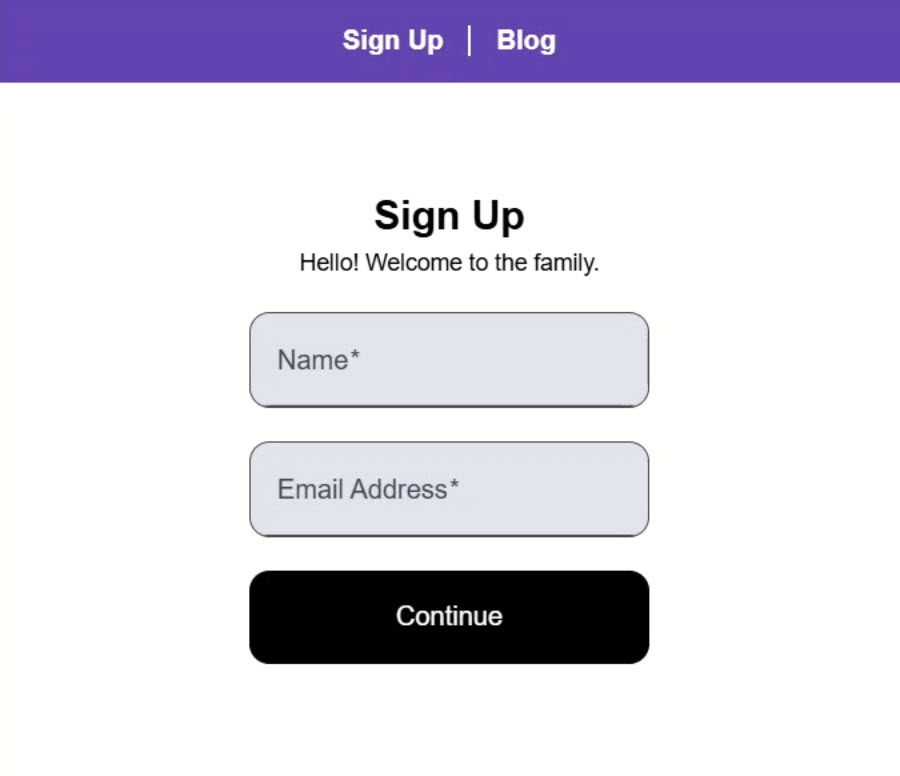
We need to add a visual container around the contents of this form.
We’ll add these styles in the the stylesheet for this sign-up form component.
Now, in order to apply styles to the root element of this component, we’ll use the :host pseudo class:
:host {
}
This is how we access the root element for our styles.
Now let’s add a border to this element:
:host {
border: solid 2px;
}
Ok, let’s save and see how it looks:
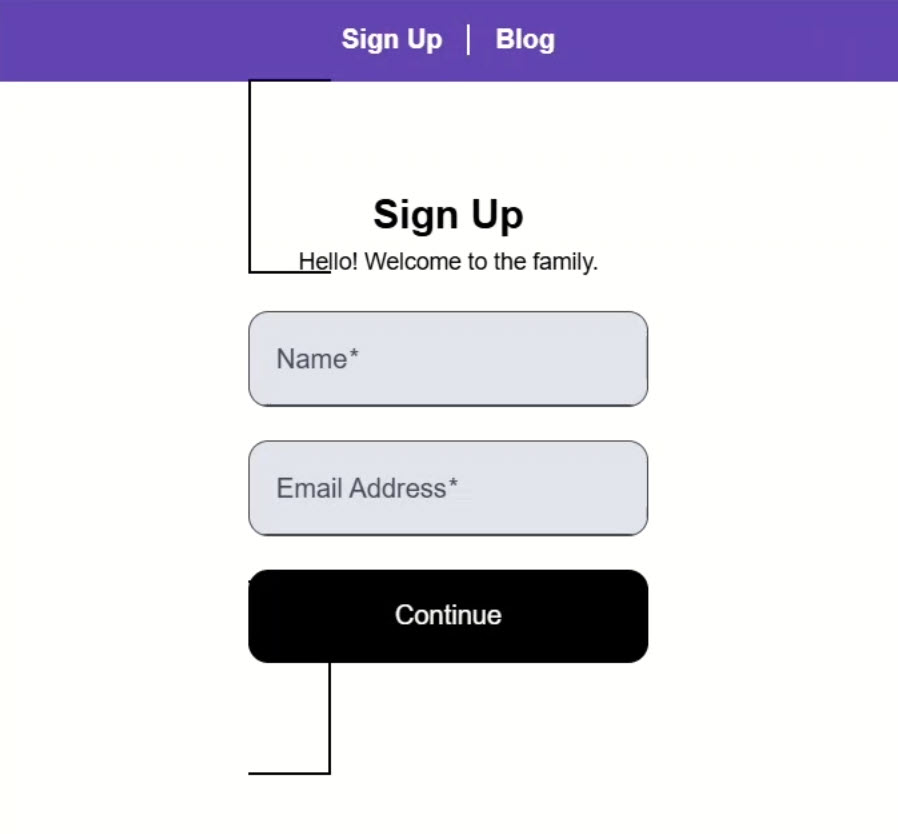
Well, that doesn’t look like a border now does it?
This is because, since this Angular component uses a custom element for its selector, the browser knows nothing about it:
@Component({
selector: 'app-sign-up-form',
...
})
This means there’s no vendor specific style information, so it’s essentially rendered inline.
So, let’s add display to the styles for this element:
:host {
display: block;
}
Let’s save and check it out now:
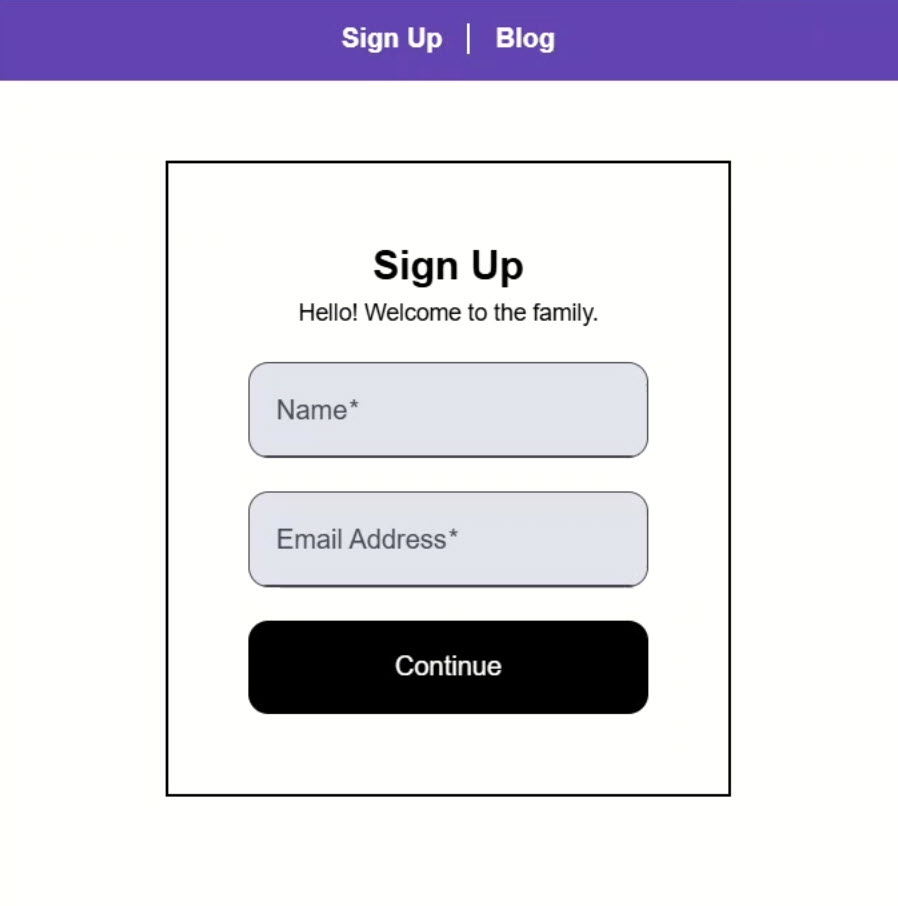
Nice, now that’s looking more like we’d expect right?
Ok, now let’s round the corners a little and give it a background color too:
:host {
display: block;
border-radius: 0.375em;
background-color: #eee;
}
Ok, let’s see how it looks now:
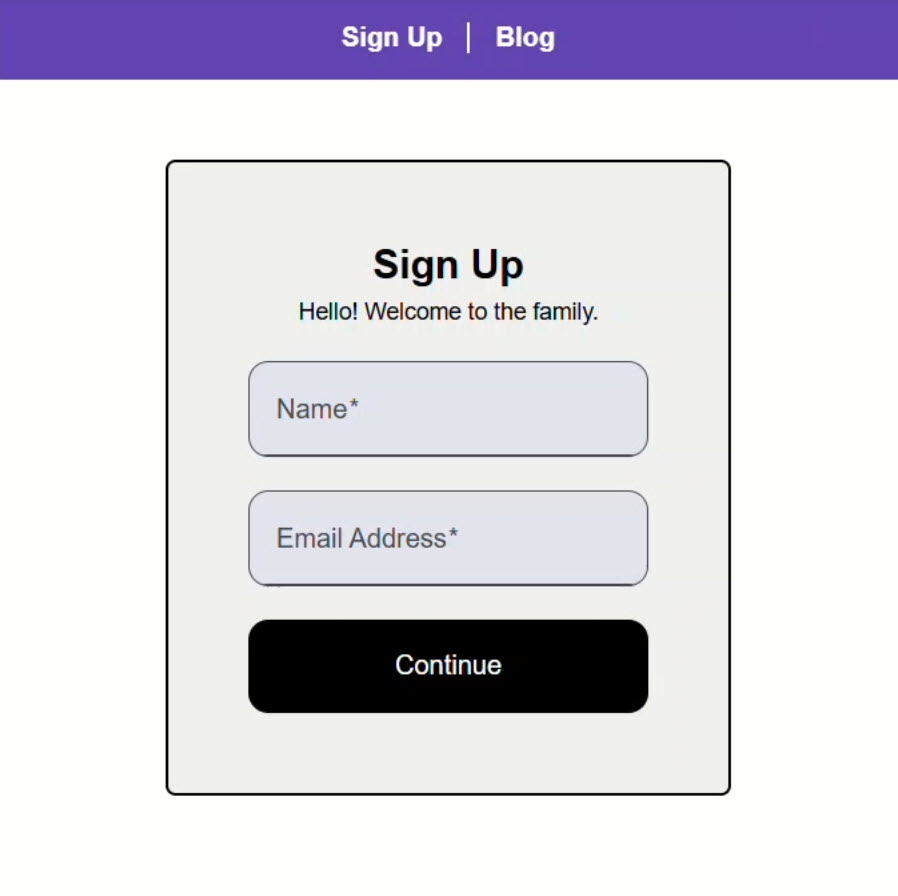
Well, that’s definitely looking better now right?
So we can use this :host pseudo class to style the root element of our component, but this also means that these styles will always apply when this component is used.
This may not be exactly what we want.
Like here in our blog where the form opens within a modal:
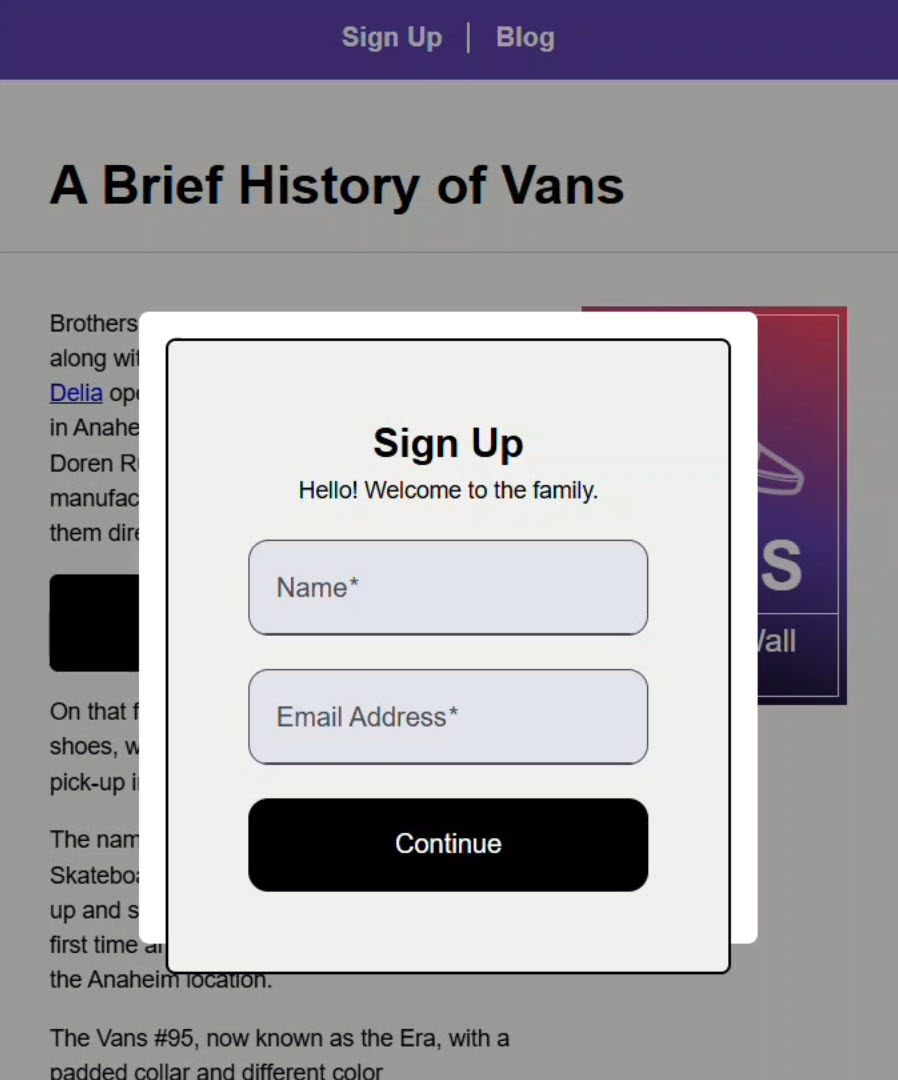
We don’t want the border or the background color here.
So how can we handle this?
Enhancing Flexibility: Conditional Styling with :host
Well, we can actually use a class in conjunction with the :host pseudo class.
To do this, let’s first add a class to the sign-up form element in the home.component.html file, let’s call it “contained”:
<app-sign-up-form class="contained"></app-sign-up-form>
Ok, now back in our stylesheet, we just need to add this class selector within parens:
:host(.contained) {
border: solid 2px;
display: block;
border-radius: 0.375em;
background-color: #eee;
}
So now these styles will only apply when this class has been added to the host element.
Let’s save and check it out:
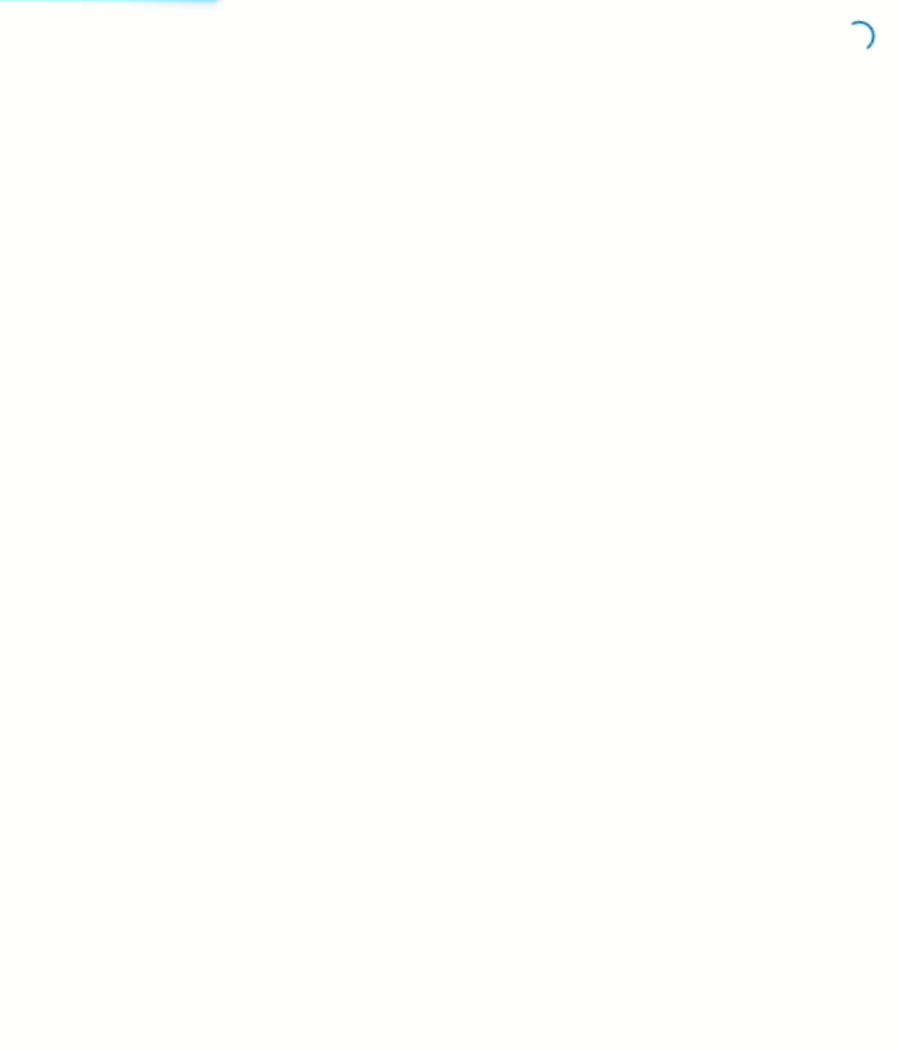
Nice, there’s no longer a container in the modal since this instance doesn’t have our new “contained” class, and for the usage in the “Sign-up” page, we still have the container styles since we added the class.
So, this concept definitely comes in handy in certain situations, but what if we decide that we want every single instance of this form to get the container styles except when placed within a modal?
Do we always have to add this “contained” class?
Nope, we have a better way.
We can use the :host-context pseudo class instead.
Context-Aware: Dynamically Adjusting Styles with :host-context
To start we can remove the “contained” class concept that we just added.
:host {
border: solid 2px;
display: block;
border-radius: 0.375em;
background-color: #eee;
}
Now these styles will apply to all instances of this component again.
Now let’s remove the styles that we don’t want in modals.
We’ll use the :host-context pseudo class for this.
We’ll need to provide a selector to use for context.
In this case, we’ll use the app-modal
element selector:
:host-context(app-modal) {
}
So, any time the app-sign-up-form
element finds itself nested within the app-modal
element, this selector will match.
Now, let’s remove the background color, and let’s also remove the border in this scenario:
:host-context(app-modal) {
background-color: unset;
border: none;
}
Ok, that should do it, let’s save and take a look:
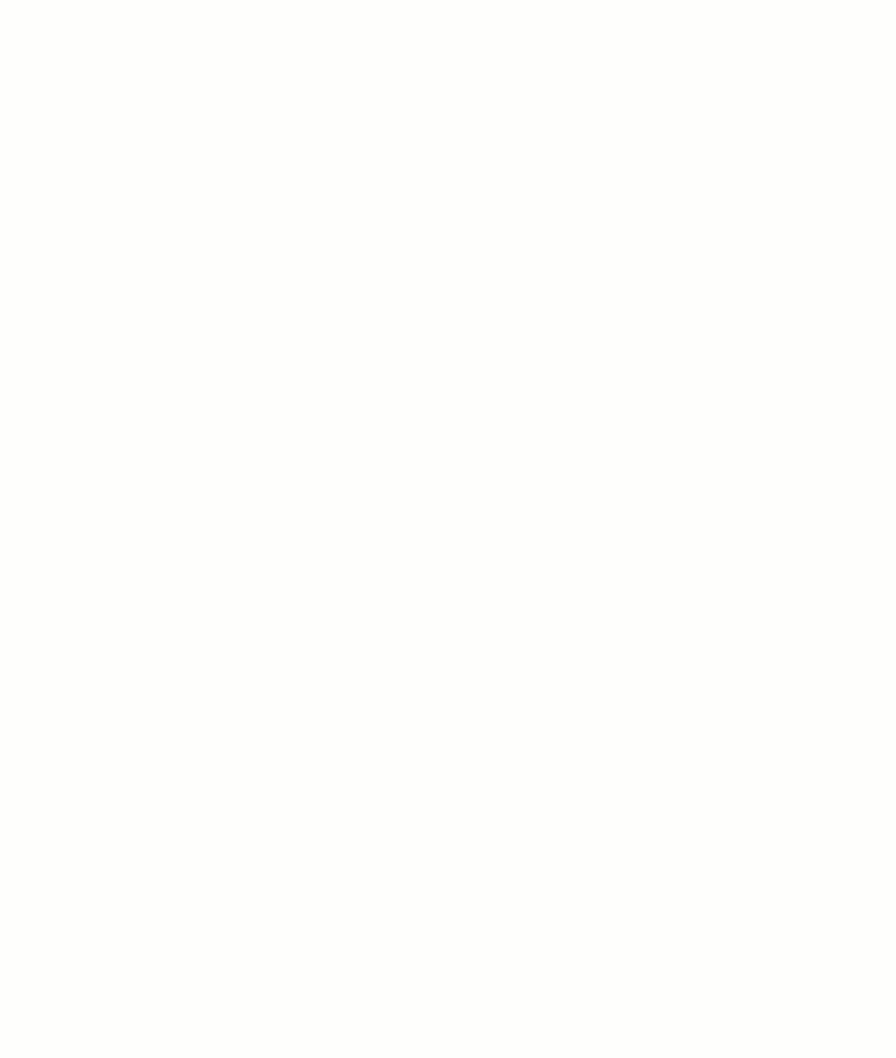
Ok, the sign-up page form looks good with the container, and when we switch over to the modal, we see that the container is no longer there.
So now, any time the component is added outside of a modal, it will get the container styles.
But, any time it is added within the modal, these container styles will be removed automatically without any extra effort.
Conclusion: Smart, Adaptive Component Styling
So now we’ve seen how :host helps us style a component’s root element and how :host-context allows us to apply styles based on where a component is used.
Understanding and using these techniques will make your Angular components more flexible, maintainable, and visually consistent across your app.
If you found this helpful you may also want to check out my course all about styles in Angular.
Also, don’t forget to check out my other Angular tutorials for more tips and tricks!
Additional Resources
- My course: “Styling Angular Applications”
- Angular styling components documentation
- The :host pseudo class
- The :host-context pseudo class
Want to See It in Action?
Check out the demo code and examples of these techniques in the Stackblitz example below. If you have any questions or thoughts, don’t hesitate to leave a comment.